Connect Dynamics 365 from Console Application using C#.NET
We can use organization service end point to connect online Dynamics 365 entities from Console application using C#.
Lets see how to do that,
Step-1 : Create Console App and add the code below in your code. Update your username and password and org.
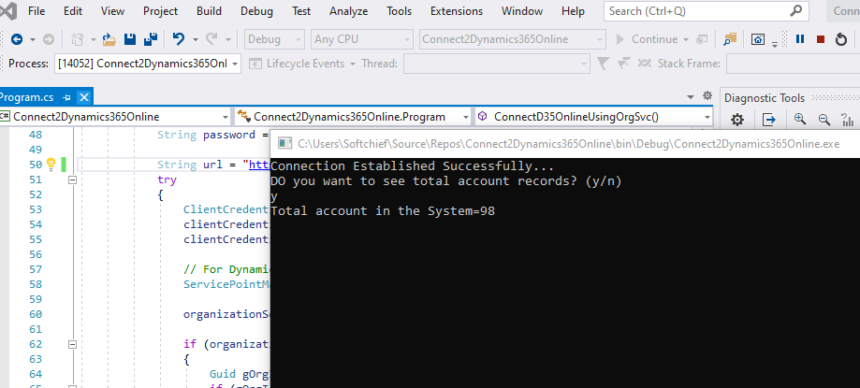
using System; using System.Collections.Generic; using System.Linq; using System.Text; //reuire namespaces using Microsoft.Xrm.Sdk; using Microsoft.Xrm.Sdk.Client; using Microsoft.Crm.Sdk.Messages; using System.Net; using System.ServiceModel.Description; using Microsoft.Xrm.Sdk.Query; namespace Connect2Dynamics365Online { class Program { static void Main(string[] args) { //Create IOrgannization Service Object IOrganizationService service = ConnectD35OnlineUsingOrgSvc(); if (service != null) { Console.WriteLine("DO you want to see total account records? (y/n)"); var response = Console.ReadLine(); if (response != "y") return; //code your logic here //Get count of contacts in the system QueryExpression qe = new QueryExpression("account"); EntityCollection enColl = service.RetrieveMultiple(qe); Console.WriteLine("Total account in the System=" + enColl.Entities.Count); Console.ReadLine(); } } public static IOrganizationService ConnectD35OnlineUsingOrgSvc() { IOrganizationService organizationService = null; String username = "<username"; String password = "<your password>"; String url = "https://<org>.api.crm.dynamics.com/XRMServices/2011/Organization.svc"; try { ClientCredentials clientCredentials = new ClientCredentials(); clientCredentials.UserName.UserName = username; clientCredentials.UserName.Password = password; // For Dynamics 365 Customer Engagement V9.X, set Security Protocol as TLS12 ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls12; organizationService = (IOrganizationService)new OrganizationServiceProxy(new Uri(url), null, clientCredentials, null); if (organizationService != null) { Guid gOrgId = ((WhoAmIResponse)organizationService.Execute(new WhoAmIRequest())).OrganizationId; if (gOrgId != Guid.Empty) { Console.WriteLine("Connection Established Successfully..."); } } else { Console.WriteLine("Failed to Established Connection!!!"); } } catch (Exception ex) { Console.WriteLine("Exception occured - " + ex.Message); } return organizationService; } } }
Step-2 : It requires assemblies of SDK and you can install using Manage Nuget package. Click on Manage Nuget Package and search “Microsoft.crmsdk.Coreassemblies” and install.
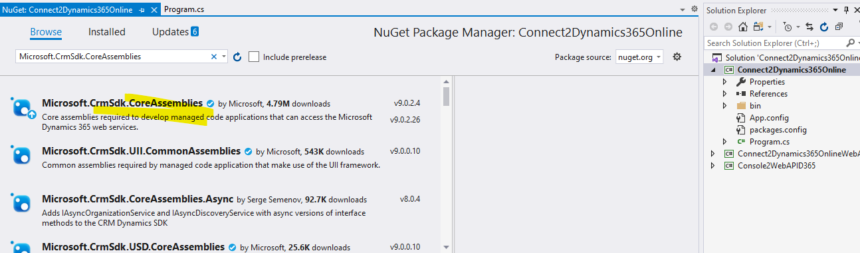
Then build it and it works.
No comments:
Post a Comment